Skills
Where Skill Meets Passion
Programming
Programming is the process of giving precise instructions to computers, enabling them to execute tasks ranging from developing software to designing websites and building applications. Computers play a vital role in contemporary life because they excel at large-scale data processing, perform complex calculations with speed and accuracy, and automate repetitive actions, thereby increasing efficiency and productivity across various industries.
Cyber Security
Cybersecurity is the practice of safeguarding computer systems, network, and confidential data from unauthorized access, making it a crucial practice in this digital era, where the proliferation of cyber threats are ever-increasing. To combat these threats, cybersecurity must remain perpetually up-to-date on new attack vectors and the technologies employed in such cyber attacks because cybercrime is one of the most rapidly evolving industries.
Web Development
Web development is the process of creating and maintaining websites and web applications. It involves a range of skills, including HTML, CSS, JavaScript, and server-side programming languages. Web developers design, code, and optimize websites to ensure they are functional, user-friendly, fast, and visually appealing. Web development is crucial for our online experiences, from e-commerce sites to social media platforms.
Linux
Linux is a remarkable free and open-source operating system that stands as a beacon of innovation in the tech world. Beyond being a powerful and highly customizable alternative to proprietary systems like Windows and MacOS, Linux is renowned for its exceptional stability, robust security, and unmatched flexibility. This makes it the top choice for a multitude of applications, from servers to supercomputers. What truly sets Linux apart is its vibrant and collaborative community, comprising developers and enthusiasts, collaborating together to improve the Linux kernel. The result is a diverse array of linux distributions, each tailored to specific needs and preferences, including renowned options like Ubuntu, Mint, Fedora, Debian, Kali, Tails, and Whonix.
Terminal
The terminal is a powerful text-based interface that forms the core of the Linux operating system, often referred to as the command line or shell. It offers users with a profound level of control, flexibility, and direct influence over the computer’s inner systems, enabling them to efficiently and precisely perform a wide range of tasks. By executing commands in the terminal, users can seamlessly transition from general tasks, such as file management and package installation, to what genuinely makes the Linux terminal invaluable: creating custom scripts to automate repetitive tasks, system configuration, software development, and more. The power of the Linux terminal lies in its efficiency, automation capabilities, and the vast number of command-line tools available.
Colemak-DH
The Colemak-DH keyboard layout is considered a superior alternative to the traditional QWERTY layout due to its optimized key placement, which promotes a more comfortable and efficient typing experience. QWERTY, designed in 1870, can lead to hand strain and reduced typing efficiency because of its legacy design aimed at slower typing to prevent typewriter jamming. Colemak-DH minimizes arm and wrist movement, with the primary motion in the fingers, making it an ergonomic choice for modern typing needs.
My Story
With a deep-rooted passion for the IT field, I find solace and purpose in the world of programming and cybersecurity. Growing up in a tech-savvy environment thanks to my father’s affinity for the newest tech gadgets, I embarked on this challenging yet exhilarating journey from a young age.
I am a 21-year-old working in Japan, and in my spare time I enjoy studying about IT. My everlasting ambition of becoming a software engineer one day fuels me through the challenging moments. I’ve self-taught myself everything in IT, a path that many wouldn’t dare to take, but I’ve never regretted this choice. This is because I deeply believe that traditional educational methods fall short in keeping pace with the ever-changing landscape of technology as information from books or courses often lags behind, massively reducing its practicality by the time its accessed and learnt by individuals. This is particularly evident in fields such as cyber security where new threats emerge 24/7.
Many compare the IT field to counting all the sand on a beach because its such a time-consuming journey. Nevertheless, I find the process of learning new things exhilarating, and I’d never get tired of it. I enjoy the effort and challenges that this field brings.
I am a 21-year-old working in Japan, and in my spare time I enjoy studying about IT. My everlasting ambition of becoming a software engineer one day fuels me through the challenging moments. I’ve self-taught myself everything in IT, a path that many wouldn’t dare to take, but I’ve never regretted this choice. This is because I deeply believe that traditional educational methods fall short in keeping pace with the ever-changing landscape of technology as information from books or courses often lags behind, massively reducing its practicality by the time its accessed and learnt by individuals. This is particularly evident in fields such as cyber security where new threats emerge 24/7.
Many compare the IT field to counting all the sand on a beach because its such a time-consuming journey. Nevertheless, I find the process of learning new things exhilarating, and I’d never get tired of it. I enjoy the effort and challenges that this field brings.
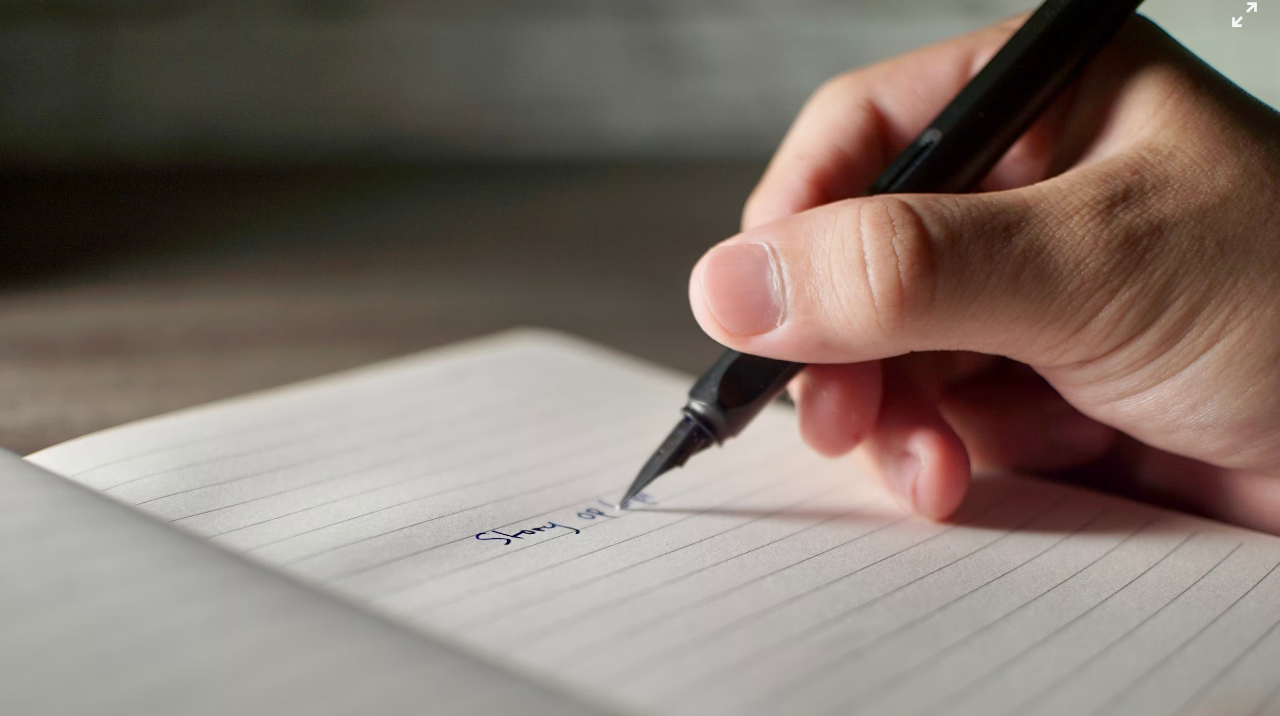
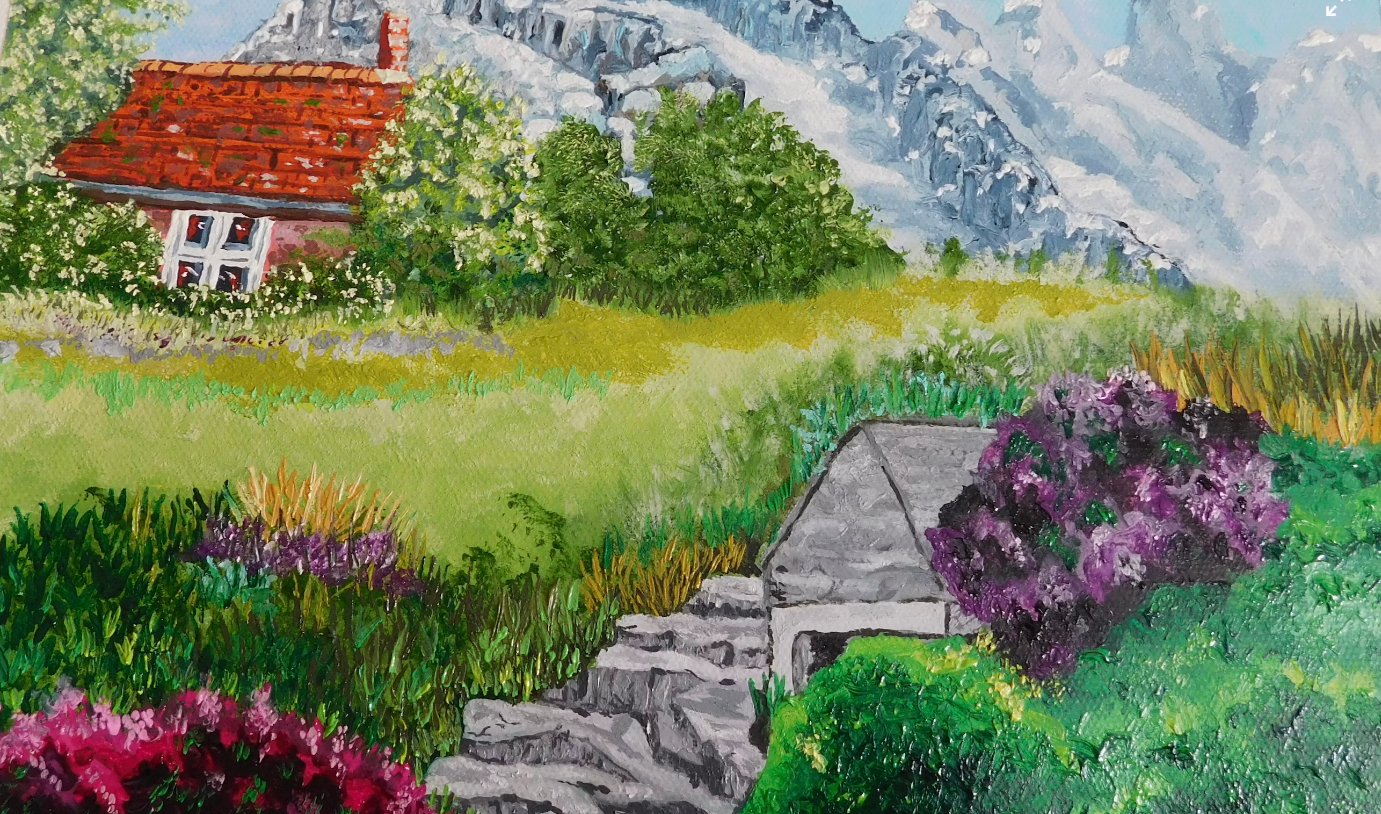
Python AES Military Grade Encryption
from cryptography.hazmat.backends import default_backend
from cryptography.hazmat.primitives.ciphers import Cipher, algorithms, modes
from base64 import urlsafe_b64encode, urlsafe_b64decode
import os
def generate_key():
return os.urandom(32)
def pad(message):
block_size = 16
padding = block_size - len(message) % block_size
return message + bytes([padding] * padding)
def unpad(message):
padding = message[-1]
return message[:-padding]
def encrypt(message, key):
key = urlsafe_b64decode(key) if key else generate_key()
message = pad(message)
cipher = Cipher(algorithms.AES(key), modes.ECB(), backend=default_backend())
encryptor = cipher.encryptor()
ciphertext = encryptor.update(message) + encryptor.finalize()
return urlsafe_b64encode(ciphertext), key
def decrypt(ciphertext, key):
try:
key = urlsafe_b64decode(key)
cipher = Cipher(algorithms.AES(key), modes.ECB(), backend=default_backend())
decryptor = cipher.decryptor()
decrypted_message = decryptor.update(urlsafe_b64decode(ciphertext)) + decryptor.finalize()
return unpad(decrypted_message).decode('utf-8')
except Exception as e:
return "Error"
# User input
while True:
operation = input("Encrypt or Decrypt [e/d]? ").lower()
if operation == 'e':
message = input("Enter the message: ")
encrypted_message, key = encrypt(message.encode('utf-8'), None)
print("Encrypted message:", encrypted_message.decode('utf-8'))
print("Decryption key:", urlsafe_b64encode(key).decode('utf-8'), "\n")
elif operation == 'd':
ciphertext = input("Encrypted message: ")
key = input("Decryption key: ")
decrypted_message = decrypt(ciphertext, key)
print(f'Recovered message: "{decrypted_message}"\n') if decrypted_message != "Error" else print("Error: Incorrect message & key pair\n")
else:
print('Error: Invalid command. Choose "e" or "d"\n')
C++ Tic-tac-toe Game
#include
using namespace std;
void DisplayGrid(string grid[3][3]);
void PlayerAction(string grid[3][3], string turn, int moves);
bool CheckInput(string grid[3][3], string turn, int moves);
bool GameFinished(string grid[3][3], string turn, int moves);
bool PlayAgain();
const string white = "⚪", black = "⚫";
string action;
int main(){
cout<<"Tic-tac-toe Game: Select location to fill from A1-C3 and be the first one to get 3 rows! Enter 'r' to restart the game. ";
do{
string grid[3][3] = { //format = [vertical][horizontal]
{"A1", "B1", "C1"},
{"A2", "B2", "C2"},
{"A3", "B3", "C3"},
};
string turn = white;
int moves = 0;
action.clear();
DisplayGrid(grid);
PlayerAction(grid, turn, moves);
}while(PlayAgain());
return 0;
}
void DisplayGrid(string grid[3][3]){ //display game grid
cout<<"\nPlayers: ⚪ | ⚫\n\n";
cout<<" | | "<>again; cin.ignore();
}while (again != 'y' && again != 'n');
cout<<"\n******"<
The Art of Programming
Programming is a fusion of creativity and logic, where innovative ideas can transform into a software with just lines of code. My ambition is to unlock the immense potential of the digital world. As a full-stack developer proficient in Python for back-end development and HTML & CSS for front-end designing, I strive to unite the rationality of programming with the creativity in designing.
Python, known for its high versatility, enables me to turn intricate algorithms and innovative ideas to life. HTML forms the foundation of websites, shaping the structure, whilst CSS adds the aesthetics to a HTML code, transforming it into a visually appealing web design.
Python, known for its high versatility, enables me to turn intricate algorithms and innovative ideas to life. HTML forms the foundation of websites, shaping the structure, whilst CSS adds the aesthetics to a HTML code, transforming it into a visually appealing web design.
Why I Chose Python
Python serves as an introductory programming language for many developers and is widely regarded as one of the most versatile programming languages available. Sometimes referred to as a “general-purpose language,” Python’s flexibility enables the creation of diverse programs. Its popularity stems from its accessibility, thanks to a vast online community and numerous high quality Python courses on YouTube, which means anyone can learn for free. Its open-source nature encourages collaborative innovation, and its large community facilitates rapid development. As the saying goes, “A community of specialists can achieve way more than any single company.”
Python excels most in scientific data analysis. However, it is often utilized in various applications such as web development, software development, task automation, machine learning, artificial intelligence, and data visualization. Despite Python being the most popular programming language, execution speed and memory consumption remain as significant drawbacks compared to some other languages. This means that Python is not suitable for graphic-intensive programs like games.
Python excels most in scientific data analysis. However, it is often utilized in various applications such as web development, software development, task automation, machine learning, artificial intelligence, and data visualization. Despite Python being the most popular programming language, execution speed and memory consumption remain as significant drawbacks compared to some other languages. This means that Python is not suitable for graphic-intensive programs like games.
Quotes Only IT Specialists Would Understand
IT Specialist Quotes
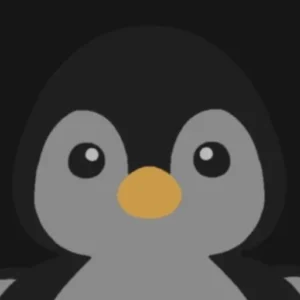
Ken Sakura
- "Explaining IT to non-techies is like teaching quantum physics to a goldfish – they nod politely, but you know it's a lost cause."
- "In IT, we don't sleep; we enter a state of low-power mode to process updates."
- "Working in IT is like juggling with Rubik's cubes – just when you think you've got one side figured out, five more pop up."
- "Saying you know everything in IT is like claiming you've read every book in the library – impossible and slightly absurd."
- "Sure, I'll become a coding prodigy as soon as I finish rewriting the code of reality."